Black Lives Matter.
Support the NAACP Legal Defense Fund or the ACLU.
color2k
a color parsing and manipulation lib served in roughly 2kB or less (2.8kB to be more precise)
color2k is a color parsing and manipulation library with the objective of keeping the bundle size as small as possible while still satisfying all of your color manipulation needs in an sRGB space.
The full bundle size is currently 2.8kB but gets as small as 2.1k with tree shaking.
Size comparison
lib | size |
---|---|
chroma-js | 13.7kB |
polished | 11.2kB |
color | 7.6kB |
tinycolor2 | 5kB |
color2k | 2.8kB π |
π Compare tree-shaken bundle outputs on bundlejs.com
Installation
Install with a package manager such as npm
npm i color2k
OR use with skypack.dev in supported environments (e.g. deno, modern browsers).
import { darken, transparentize } from 'https://cdn.skypack.dev/color2k?min';
Usage
import { darken, transparentize } from 'color2k';
// e.g.
darken('blue', 0.1);
transparentize('red', 0.5);
How so small?
There are two secrets that keep this lib especially small:
- Simplicity β only handles basic color manipulation functions
- Less branching in code β only support two color models as outputs, namely
rgba
andhsla
Why only rgba
and hsla
as outputs?
This lib was created with the use case of CSS-in-JS in mind. At the end of the day, all that matters is that the browser can understand the color string you gave it as a background-color
.
Because only those two color models are supported, we don't have to add code to deal with optional alpha channels or converting to non-browser supported color models (e.g. CMYK).
We believe that this lib is sufficient for all of your color manipulation needs. If we're missing a feature, feel free to open an issue π.
Credits
Heavy credits goes to polished.js and sass. Much of the implementation of this lib is copied from polished!
CodeSandbox Demo
Try out color2k in CodeSandbox
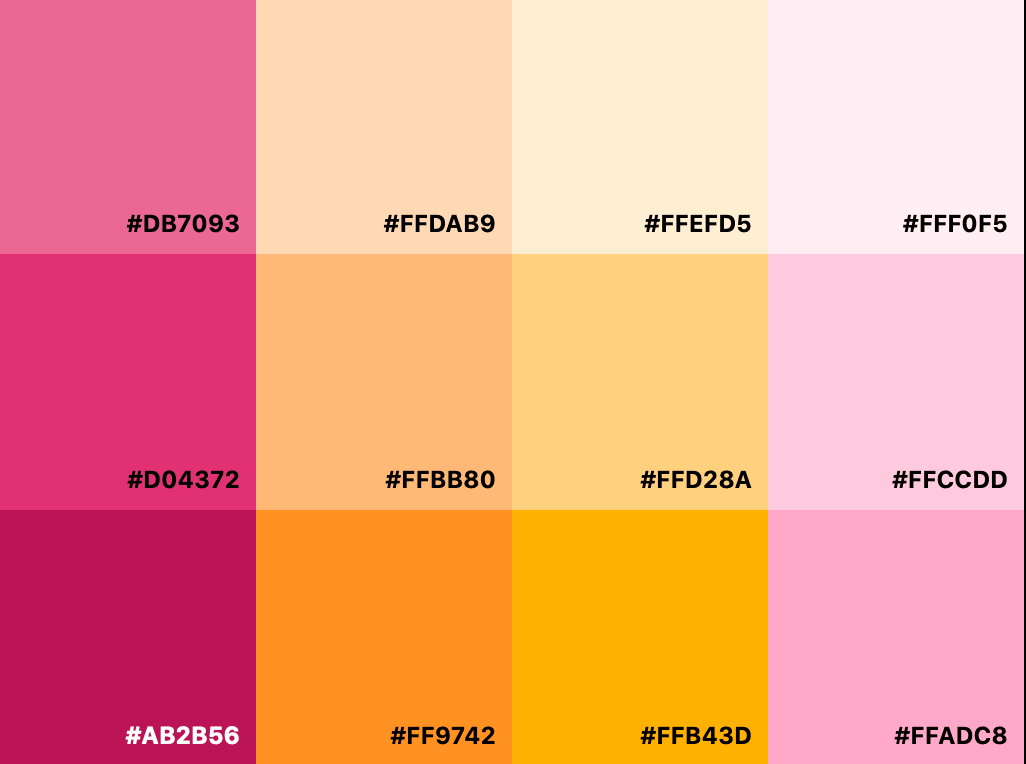
adjustHue(color, degrees): string
Adjusts the current hue of the color by the given degrees. Wraps around when over 360.
Param | Type | Description |
---|---|---|
color | string | input color |
degrees | number | degrees to adjust the input color, accepts degree integers (0 - 360) and wraps around on overflow |
darken(color, amount): string
Darkens using lightness. This is equivalent to subtracting the lightness from the L in HSL.
Param | Type | Description |
---|---|---|
color | string | |
amount | number | The amount to darken, given as a decimal between 0 and 1 |
desaturate(color, amount): string
Desaturates the input color by the given amount via subtracting from the s
in hsla
.
Param | Type | Description |
---|---|---|
color | string | |
amount | number | The amount to desaturate, given as a decimal between 0 and 1 |
getContrast(color1, color2): number
Returns the contrast ratio between two colors based on W3's recommended equation for calculating contrast.
Param | Type | |
---|---|---|
color1 | string | |
color2 | string |
getLuminance(color): number
Returns a number (float) representing the luminance of a color.
Param | Type | |
---|---|---|
color | string |
getScale(colors): (n: number) => string
Given a series colors, this function will return a scale(x)
function that
accepts a percentage as a decimal between 0 and 1 and returns the color at
that percentage in the scale.
const scale = getScale('red', 'yellow', 'green');
console.log(scale(0)); // rgba(255, 0, 0, 1)
console.log(scale(0.5)); // rgba(255, 255, 0, 1)
console.log(scale(1)); // rgba(0, 128, 0, 1)
If you'd like to limit the domain and range like chroma-js, we recommend wrapping scale again.
const _scale = getScale('red', 'yellow', 'green');
const scale = x => _scale(x / 100);
console.log(scale(0)); // rgba(255, 0, 0, 1)
console.log(scale(50)); // rgba(255, 255, 0, 1)
console.log(scale(100)); // rgba(0, 128, 0, 1)
Param | Type | |
---|---|---|
colors | string[] |
guard(low, high, value): number
A simple guard function:
Math.min(Math.max(low, value), high)
Param | Type | |
---|---|---|
low | number | |
high | number | |
value | number |
hasBadContrast(color, standard, background): boolean
Returns whether or not a color has bad contrast against a background according to a given standard.
Param | Type | |
---|---|---|
color | string | |
standard | 'decorative' | 'readable' | 'aa' | 'aaa' = 'aa' | |
background | string = '#fff' |
hsla(hue, saturation, lightness, alpha): string
Takes in hsla parts and constructs an hsla string
Param | Type | Description |
---|---|---|
hue | number | The color circle (from 0 to 360) - 0 (or 360) is red, 120 is green, 240 is blue |
saturation | number | Percentage of saturation, given as a decimal between 0 and 1 |
lightness | number | Percentage of lightness, given as a decimal between 0 and 1 |
alpha | number | Percentage of opacity, given as a decimal between 0 and 1 |
lighten(color, amount): string
Lightens a color by a given amount. This is equivalent to
darken(color, -amount)
Param | Type | Description |
---|---|---|
color | string | |
amount | number | The amount to darken, given as a decimal between 0 and 1 |
mix(color1, color2, weight): string
Mixes two colors together. Taken from sass's implementation.
Param | Type | |
---|---|---|
color1 | string | |
color2 | string | |
weight | number |
opacify(color, amount): string
Takes a color and un-transparentizes it. Equivalent to
transparentize(color, -amount)
Param | Type | Description |
---|---|---|
color | string | |
amount | number | The amount to increase the opacity by, given as a decimal between 0 and 1 |
parseToHsla(color): [number, number, number, number]
Parses a color in hue, saturation, lightness, and the alpha channel.
Hue is a number between 0 and 360, saturation, lightness, and alpha are decimal percentages between 0 and 1
Param | Type | |
---|---|---|
color | string |
parseToRgba(color): [number, number, number, number]
Parses a color into red, gree, blue, alpha parts
Param | Type | Description |
---|---|---|
color | string | the input color. Can be a RGB, RBGA, HSL, HSLA, or named color |
readableColor(color): string
Returns black or white for best contrast depending on the luminosity of the given color.
Param | Type | |
---|---|---|
color | string |
readableColorIsBlack(color): boolean
An alternative function to readableColor
. Returns whether or not the
readable color (i.e. the color to be place on top the input color) should be
black.
Param | Type | |
---|---|---|
color | string |
rgba(red, green, blue, alpha): string
Takes in rgba parts and returns an rgba string
Param | Type | Description |
---|---|---|
red | number | The amount of red in the red channel, given in a number between 0 and 255 inclusive |
green | number | The amount of green in the red channel, given in a number between 0 and 255 inclusive |
blue | number | The amount of blue in the red channel, given in a number between 0 and 255 inclusive |
alpha | number | Percentage of opacity, given as a decimal between 0 and 1 |
saturate(color, amount): string
Saturates a color by converting it to hsl
and increasing the saturation
amount. Equivalent to desaturate(color, -amount)
Param | Type | Description |
---|---|---|
color | string | Input color |
amount | number | The amount to darken, given as a decimal between 0 and 1 |
toHex(color): string
Takes in any color and returns it as a hex code.
Param | Type | |
---|---|---|
color | string |
toHsla(color): string
Takes in any color and returns it as an hsla string.
Param | Type | |
---|---|---|
color | string |
toRgba(color): string
Takes in any color and returns it as an rgba string.
Param | Type | |
---|---|---|
color | string |
transparentize(color, amount): string
Takes in a color and makes it more transparent by convert to rgba
and
decreasing the amount in the alpha channel.
Param | Type | Description |
---|---|---|
color | string | |
amount | number | The amount to increase the transparency by, given as a decimal between 0 and 1 |